Capacitor + Supabase + Social Auth + React In-App
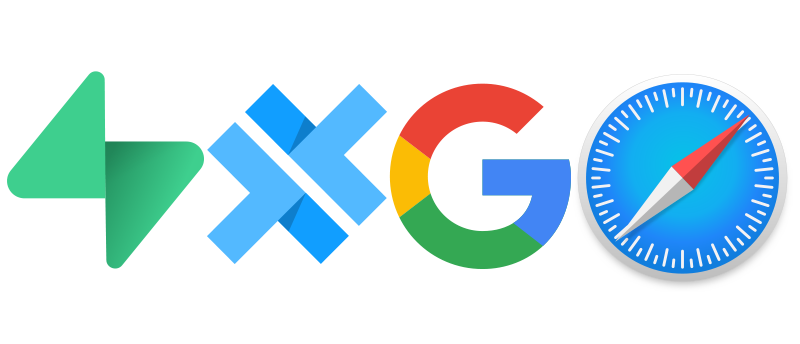
In my previous, extremely long tutorial post I walked you through how to get a simple React app to use Supabase Social authentication to work within a Capacitor app. Phew that was a lot of name dropping sorry.
This post will be much much shorter, the goal in this second part is to explain how to extend upon the work we previously did to use the in-app browser experience. The in-app browser the best way to use the social logins as it gives the "normal" experience users expect when logging in this way but more critically it does not depend on the users default browser.
Setup
If you followed along with the previous post you should have a reasonably working native application that can use Google auth flow to authenticate with Supabase. Once you have that working (be sure to have Safari as your default browser) you should be able to get started here.
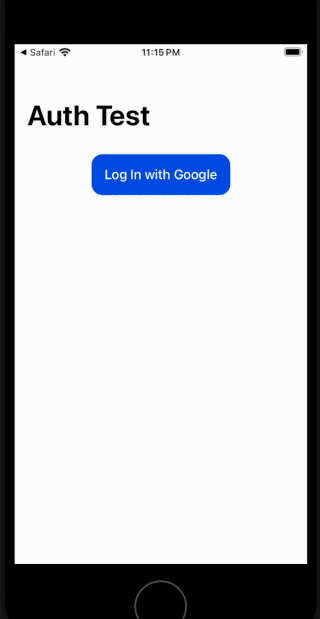
The three problems we have:
- It depends on the current default browser, this could be something like Brave or Orion which do not support deep links (at least as of this writing and in this situation)
- It doesn't feel "normal" for a user using your native app to bounce out of the app for authentication.
- The page that you authenticated with remains there in the browser, the user might get confused or just feel your app is sloppy when it leaves a trail like that.
Prevent Redirect
The first thing we need to do is stop Supabase from automatically launching the browser when running the sign in code. Let's do this by adding the lightly documented prop called skipBrowserRedirect
to the signInWithOauth
call
components/LoginComponent.tsx
const login = () => {
supabase.auth.signInWithOAuth({
provider: 'google',
options: {
redirectTo: `${baseUrl}/logged`,
+ // Skip the Browser Redirect to manually open the browser
+ skipBrowserRedirect: true,
queryParams: {
access_type: 'offline',
prompt: 'consent',
},
},
})
}
With that addition we no longer automatically launch the browser when the user attempts to log in with this method. We have to do that manually now, but because we do it manually we have some control on how we launch that browser.
And with that we can now use the "in app browser".
In App Browser
The In-App Browser uses the built in browser engine of the devices which means it's consistent regardless of what other browser is set as the default. This also helps us use things we are know supported by these browsers, in this case Deep Linking.
To open an in-app browser in Capacitor we will use the documentation they have provided: https://capacitorjs.com/docs/apis/browser. Notice that this isn't the seemingly better named "in app" browser version 🤷.
pnpm i @capactior/browser
And let's create a new component to handle the details of this browser experience. Notice that we are no longer just writing code for a web app, we are now leaning into some of the nice things that Capacitor gives us and is able to adapt automatically based on the environment it lives in.
nativeBrowser.ts
import { Capacitor } from '@capacitor/core'
import { Browser } from '@capacitor/browser'
export const openBrowser = async (url: string) => {
// Open the browser only if we are on a native platform (in-app)
if (Capacitor.isNativePlatform()) {
await Browser.open({
url,
presentationStyle: 'popover',
})
} else {
window.location.href = url
}
}
export const closeBrowser = async () => {
if (Capacitor.isNativePlatform()) {
await Browser.close()
}
}
Notice how we have to check if we are in a native platform or web. If we are in a native environment we want to trigger this new Browser.open function call so we can designate how that experience should look.
Now back at our LoginComponent we want to trigger the openBrowser function call manually to get the authentication flow kicked off.
components/LoginComponent.tsx
const login = async () => {
+ const { data, error } = await supabase.auth.signInWithOAuth({
provider: 'google',
options: {
redirectTo: `${baseUrl}/logged`,
// Skip the Browser Redirect to manually open the browser
skipBrowserRedirect: true,
queryParams: {
access_type: 'offline',
prompt: 'consent',
},
},
})
+ if (error) {
+ console.error(error)
+ return
+ }
+ await openBrowser(data.url)
}
Obviously we could do something different with the error handling, but for now this is an example. The key is that we manually open the browser now and depending if we are in a native environment or standard browser we handle it slightly differently.
If you attempt to use the app now you'll notice that the in-app browser works and it looks nice except it sticks open. So close!
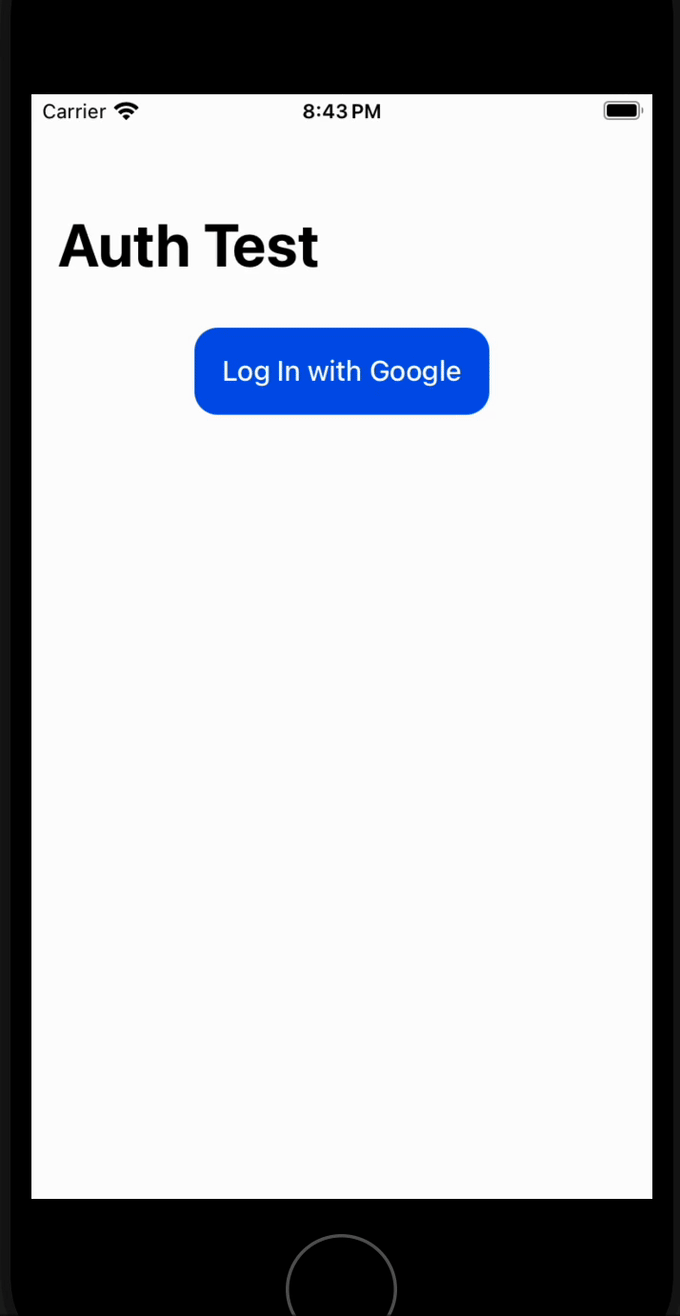
Closing the Browser
If you test this out now you'll notice that the browser just hangs open after you authenticate. Luckily we have a function (we already wrote) to close that browser and thus we just need to call that on the redirect page we built in the first post.
We know that we are redirected to the /logged
page when we authenticate, so we could brute force the browser closed by saying 'when that page is loaded, close the external browser if there is one'. That could be done with code like this:
components/LoggedIn.tsx
+ import { closeBrowser } from '../nativeBrowser'
export const LoggedIn = () => {
const history = useHistory()
const { supabase } = useSupabase()
+ useEffect(() => {
+ closeBrowser().catch(() => {
+ // nom nom nom
+ })
+ }, [])
....
// rest of the component
This would simply close the browser when this component is rendered. This would of course be kind of poor form if we wanted to use this page for anything else or if we landed on this page not from the result of logging in.
So a better way to handle this would be to move that logic to the SupabaseProvider we've created in the previous post. Here we can listen for authentication state changes and do some logic based on the "logged in" state.
components/SupabaseProvider.tsx
export const SupabaseProvider: FC<SupabaseProviderProps> = ({ children }) => {
const [supabase] = useState<SupabaseClient>(() =>
createClient(supabaseUrl, supabaseKey),
)
+ useEffect(() => {
+ const { data: authListener } = supabase.auth.onAuthStateChange(
+ (event, session) => {
+ if (event === 'SIGNED_IN' && session?.user) {
+ closeBrowser().catch(() => {
+ // nom nom nom
+ })
+ }
+ },
+ )
+ // Cleanup the listener on unmount
+ return () => {
+ authListener?.subscription.unsubscribe()
+ }
+ }, [])
...// The rest of the component
This will listen for the events emitted from the supabase auth and do logic based on that. In our case we simply want to close the browser but you could also expand this to saving the user you get back.
If you use the SupabaseProvider change make sure to remove the LoggedIn.tsx change.
Congrats you can now test the application login and it'll have the standard in-app browser experience that users are expecting.
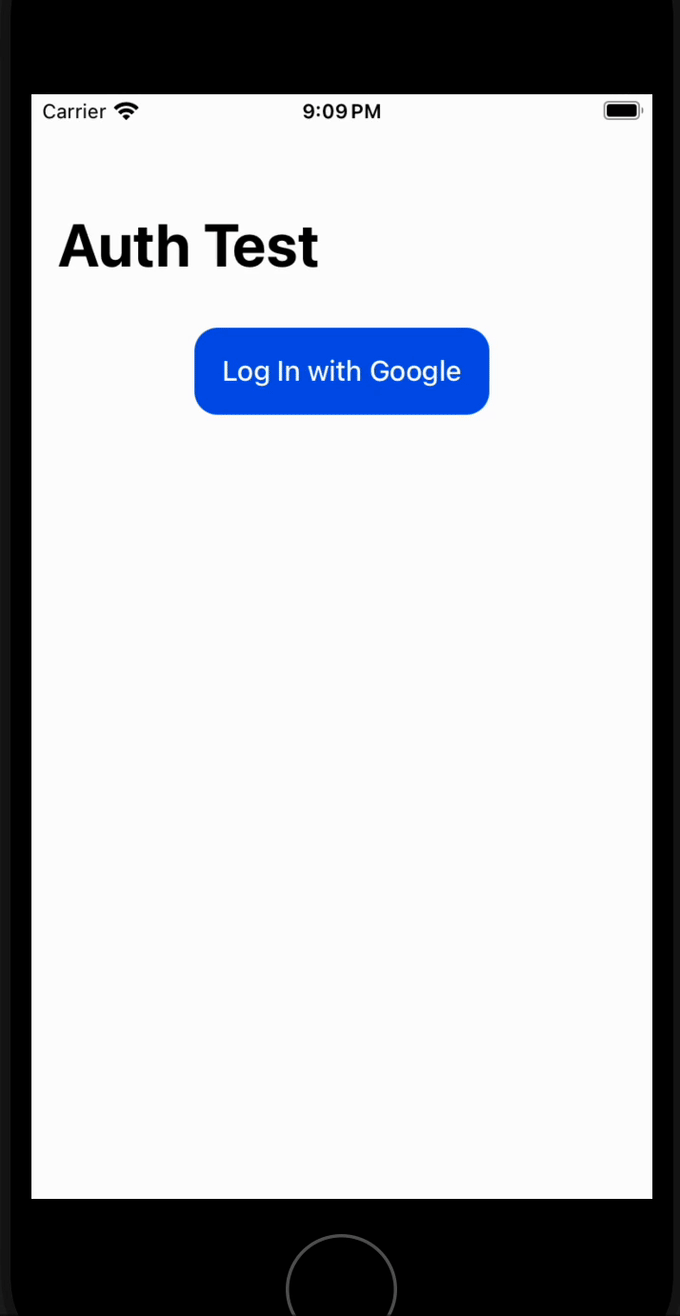
Conclusion
Congrats on getting this far! The goal of this post was to make the example app a bit more "natural" feeling but unfortunately it required the first step of "if native do X" type of checking.
I like to try and keep the code I write the same across all devices, similar to not having to use specific CSS selectors or other varieties of things that are browser specific in web development. This is a nessissary evil and by abstracting it away into the nativeBrowser.tsx
component it's at least nicer to look at and use across the app.
This only leaves one last downfall:
- You must use the "consent" variety of authentication.
I haven't yet seen the auth flow work properly even in the in-app experience if you don't use the consent flow (slower, more windows for people to click through). Maybe in the future I'll add yet more native-specific code so we can skip those screens. For now this is good enough for me and doesn't feel too janky to the user.